Here's a basic script solution that should do what you want.
I tested it on a simplified table that looks like this.
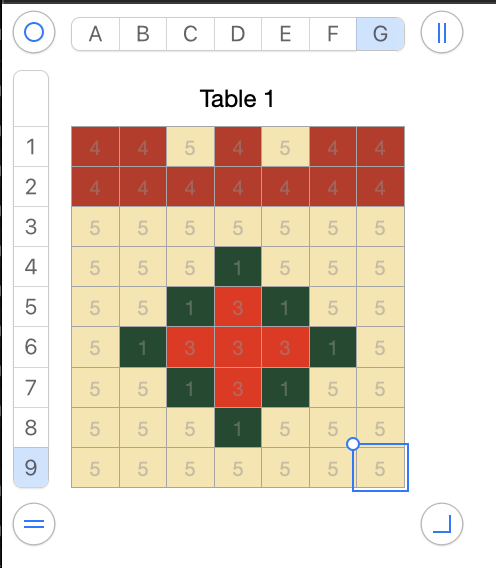
The script:
tell application "Numbers"
tell active sheet of front document
tell (first table whose class of selection range is range)
set background color of cells whose value is 1 to my hexColorToRGB("#143C23")
set background color of cells whose value is 2 to my hexColorToRGB("#20A603")
set background color of cells whose value is 3 to my hexColorToRGB("#E7000D")
set background color of cells whose value is 4 to my hexColorToRGB("#B21D1A")
set background color of cells whose value is 5 to my hexColorToRGB("#F5E09D")
end tell
end tell
end tell
property hexChrs : "0123456789abcdef"
on hexColorToRGB(h)
set h to text 2 thru -1 of h
if (count h) < 6 then my badColor(h)
set rgbColor to {}
try
repeat with i from 1 to 6 by 2
set end of rgbColor to ((my getHexVal(text i of h)) * 16 + (my getHexVal(text (i + 1) of h))) * 257
end repeat
end try
if (count rgbColor) < 3 then my badColor(h)
return rgbColor
end hexColorToRGB
on getHexVal(c)
if c is not in hexChrs then error
set text item delimiters to c
return (count text item 1 of hexChrs)
end getHexVal
on badColor(n)
display alert n & " is not a valid hex color" buttons {"OK"} cancel button "OK"
end badColor
- Copy-paste into Script Editor (in Applications > Utilities)
- Change the hex values in the five lines near the beginning of the script to suit.
- Make sure Script Editor is listed and checked at System Preferences > Security & Privacy > Privacy > Accessibility.
- Click in the table that has the codes 1 through 5 in the cells.
- Click the triangle <run> button in Script Editor.
Bonus. Below is a script that allows you to retrieve the hex value of the background color of a selected cell. So you can color a cell to taste using the inspector pane at the right, then retrieve the hex value of the cell and insert that in the script above in the line for the code (1 to 5) the you want to assume that color.
tell application "Numbers" to tell front document
set t to first table of active sheet whose class of selection range is range
set selRangeName to name of selection range of t
set bkgrRgb to background color of cell 1 of range selRangeName of t
if bkgrRgb is missing value then error "Selected cell has no background color"
set bkgrColorHex to my convertRGBColorToHexValue(bkgrRgb)
end tell
on convertRGBColorToHexValue(theRGBValues)
set theHexList to {"0", "1", "2", "3", "4", "5", "6", "7", "8", "9", "A", "B", "C", "D", "E", "F"}
set theHexValue to ""
repeat with a from 1 to count of theRGBValues
set theCurrentRGBValue to (item a of theRGBValues) div 256
if theCurrentRGBValue is 256 then set theCurrentRGBValue to 255
set theFirstItem to item ((theCurrentRGBValue div 16) + 1) of theHexList
set theSecondItem to item (((theCurrentRGBValue / 16 mod 1) * 16) + 1) of theHexList
set theHexValue to (theHexValue & theFirstItem & theSecondItem) as string
end repeat
set theHexValue to "#" & theHexValue
set the clipboard to theHexValue
return theHexValue
end convertRGBColorToHexValue
I've found that the easiest way to compare before and after colors is to duplicate the original table (click, then click the "bulls-eye" concentric circles upper left, command-c to copy, click the canvas on the sheet, and command-v to paste). Then click in that duplicated table before running the first script above (after changing the hex values in it to the new colors).
SG