Another issue is that when you launch Pages, by default, it will open the Template Chooser, and just sit there waiting for you to pick one. The application will remain open and running indefinitely in this state, until it quits by user interaction, or your script then quits it. I have included some Python code that incorporates twtwtw's AppleScript, and you can see how the Python code runs the AppleScript as a subprocess. Finally, a script completion notification will display. I have used the optional Anaconda Python syntax checker in Sublime Text 3, and there are no Python syntax issues.
I do not write Python code with great whacking gaps in the code, the following is the result of using the Python syntax facility from this editor.
#!/usr/bin/env python
# -*- coding: utf-8 -*-
import sys
import subprocess
ascmd = u"""
on run
tell application "Pages" to activate
-- add a longish delay to allow the application to start
delay 10
tell application "Pages" to quit
end run
on idle
if application "Pages" is not running then
-- the application has quit, so go to the 'on quit' handler
quit
end if
end idle
on quit
set notificationTitle to "AppleScript Quit Handler"
set notificationSubTitle to "Pages Status"
set notificationMsg to "Not Running"
display notification notificationMsg with title notificationTitle ¬
subtitle notificationSubTitle
continue quit
end quit
"""
ascmd_done = u"""
set notificationTitle to "Python/Osascript Test"
set notificationSubTitle to "Test Status"
set notificationMsg to "Finished"
display notification notificationMsg with title notificationTitle ¬
subtitle notificationSubTitle
"""
def main():
try:
result = subprocess.check_output(['osascript', '-e', ascmd])
# print("\nResult: %s\n" % result)
if 'Cancel' not in result.decode('utf-8'):
subprocess.check_output(['osascript', '-e', ascmd_done])
except subprocess.CalledProcessError as e:
print('Python error: [%d]\n%s\n' % e.returncode, e.output)
if __name__ == '__main__':
sys.exit(main())
Here is what the formatted Python script looks like:
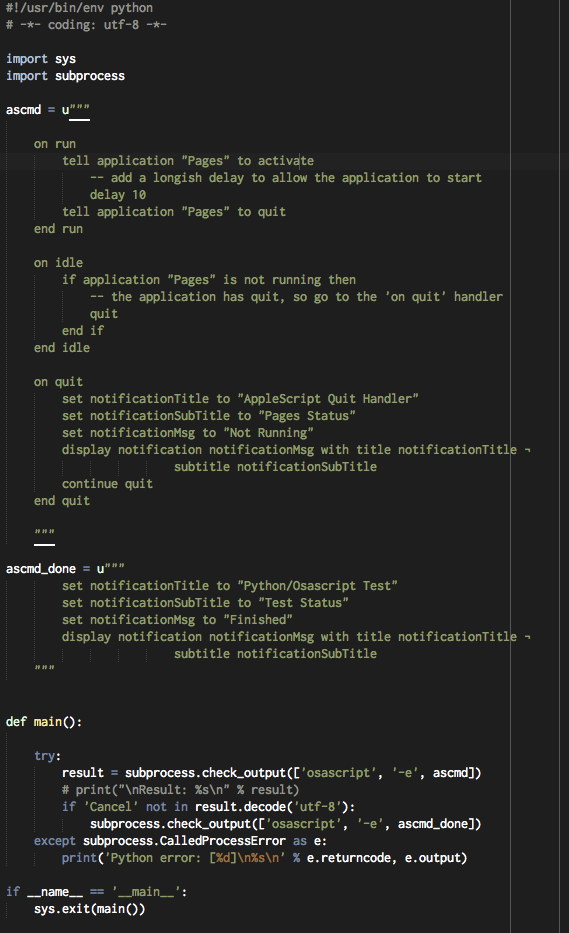