Hi Cloud4846. One option is to create a small utility to detect a depressed mouse button for use in an AppleScript. Copy and paste the following Swift code into a text editor such as Sublime Text and save it your Desktop with a .swift file extension using a name such as mouse.swift:
import Foundation
import AppKit
func TranslateButtonState(buttonState: Int) -> String {
if (buttonState == 1 << 0) { return "left"; }
if (buttonState == 1 << 1) { return "right"; }
return "none";
}
var buttonState = NSEvent.pressedMouseButtons()
print(TranslateButtonState(buttonState))
With Xcode installed compile the above code with the Swift compiler in Terminal using the following command which places the binary in /usr/local/bin:
xcrun -sdk macosx swiftc ~/Desktop/mouse.swift -o /usr/local/bin/mouse
You can test the utility in Terminal by preceding the mouse command with a delay and depressing the left or right mouse button:
sleep 1; mouse
Output returned is one of “left”, “right”, or “none”. The utility also works with a one finger or two finger mechanical depression of a trackpad for the primary and secondary click respectively.
You can use the utility in an AppleScript to display a dialog message when a mouse button is depressed, in this example the right mouse button. Keep in mind that because of AppleScript’s single-threaded nature and that the "do shell script" is in the loop with a delay, this is really only practical with short delays as longer delays necessitate holding down the mouse button longer:
tell application "System Events"
repeat 10 times
set mouseDown to (do shell script "/usr/local/bin/mouse")
if mouseDown = "right" then
key code 87
display dialog "Do you want to exit this script?" buttons {"Exit", "Continue"} default button 1
if result = {button returned:"Exit"} then
return
end if
else
delay 0.5
key code 87
delay 0.5
end if
end repeat
end tell
Rather than use a mouse button down event to trigger a dialog, you can use a key press such as a modifier key. Copy and paste the following Swift code into a text editor and save it to your Desktop with a .swift file extension using a name such as modKeys.swift:
import Foundation
import AppKit
func TranslateModifierFlags(modifierFlags: NSEventModifierFlags) -> String {
let rawModifierFlags = modifierFlags.rawValue
var pressedButtons = [String]()
if ((rawModifierFlags & NSEventModifierFlags.ControlKeyMask.rawValue) != 0) { pressedButtons.append("Control") }
if ((rawModifierFlags & NSEventModifierFlags.AlternateKeyMask.rawValue) != 0) { pressedButtons.append("Option") }
if ((rawModifierFlags & NSEventModifierFlags.ShiftKeyMask.rawValue) != 0) { pressedButtons.append("Shift") }
if ((rawModifierFlags & NSEventModifierFlags.CommandKeyMask.rawValue) != 0) { pressedButtons.append("Command") }
if (pressedButtons.count > 0) {
return pressedButtons.joinWithSeparator(" ")
}
return "none"
}
var modifierFlags = NSEvent.modifierFlags()
print(TranslateModifierFlags(modifierFlags))
With Xcode installed compile the above code with the Swift compiler in Terminal using the following command which places the binary in /usr/local/bin:
xcrun -sdk macosx swiftc ~/Desktop/modKeys.swift -o /usr/local/bin/modKeys
You can test the utility in Terminal by preceding the mouse command with a delay and depressing any of the four modifier keys Control, Option, Shift, and Command either individually or in combination:
sleep 1; modKeys
Output returned is one or more of “Control”, “Option”, “Shift”, and “Command”. An example of the utility and the Shift key used in an Applescript:
tell application "System Events"
repeat 10 times
set keyDown to (do shell script "/usr/local/bin/modKeys")
if keyDown = “Shift" then
key code 87
display dialog "Do you want to exit this script?" buttons {"Exit", "Continue"} default button 1
if result = {button returned:"Exit"} then
return
end if
else
delay 0.5
key code 87
delay 0.5
end if
end repeat
end tell
The most appropriate modifier keys to use are Shift and Option.
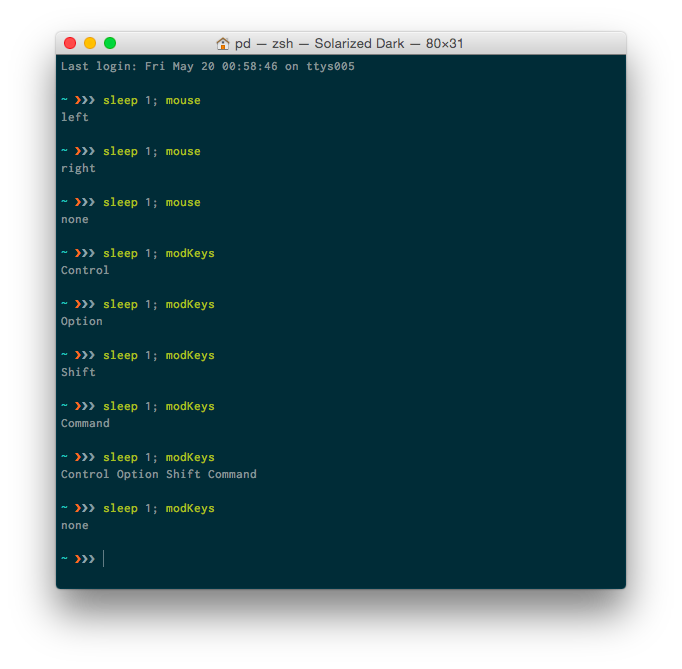
Tested with OS X Yosemite 10.10.5, Script Editor 2,7, AppleScript 2.4, Xcode 7.2.1
AppKit Framework Reference > NSEvent Class Reference
The Swift Programming Language
Introduction to AppleScript Language Guide