I suggest you look closer at the POSIX popen(3) approach in C++ to avoid the system() call. Python can write to sys.stdout with its print statement, provided you import sys:

Here is how you access the output of a Python3 program from within C++ (c++11). In this example, the ntp_time.py script is in the same location as the compiled C++ code.
C++ compiles on macOS 12.3.1 with current clang++ 13.1.6:
// clang++ -Oz -o py_out py_out.C -std=c++11
// Reference: https://stackoverflow.com/questions/44610978/popen-writes-output-of-command-executed-to-cout
#include <string>
#include <iostream>
#include <cstdlib>
#include <cstdio>
#include <array>
int main()
{
std::string command("ntp_time.py 2>&1");
std::array<char, 128> buffer;
std::string result;
std::cout << "Opening reading pipe" << std::endl;
FILE* pipe = popen(command.c_str(), "r");
if (!pipe)
{
std::cerr << "Couldn't start command." << std::endl;
return 0;
}
while (fgets(buffer.data(), 128, pipe) != NULL) {
std::cout << "Reading..." << std::endl;
result += buffer.data();
}
auto returnCode = pclose(pipe);
std::cout << result;
std::cout << returnCode << std::endl;
return 0;
}
and the ntp_time.py source tested in macOS 12.3.1 with Python.org's Python 3.10.4:
#!/usr/bin/env python3
from socket import AF_INET, SOCK_DGRAM
import socket
import struct
import time
# def getNTPTime(host="pool.ntp.org"):
def getNTPTime(host="time.nist.gov"):
port = 123
buf = 1024
address = (host, port)
msg = '\x1b' + 47 * '\0'
# reference time (in seconds since 1900-01-01 00:00:00)
TIME1970 = 2208988800 # 1970-01-01 00:00:00
# connect to server
client = socket.socket(AF_INET, SOCK_DGRAM)
client.sendto(bytes(msg.encode('utf-8')), address)
msg, address = client.recvfrom(buf)
t = struct.unpack("!12I", msg)[10]
t -= TIME1970
s = time.localtime(t)
# s = time.gmtime(t)
# s = time.ctime(t).replace(" ", " ")
return time.strftime('%FT%X %Z', s)
if __name__ == "__main__":
print("{}".format(getNTPTime()))
Result:
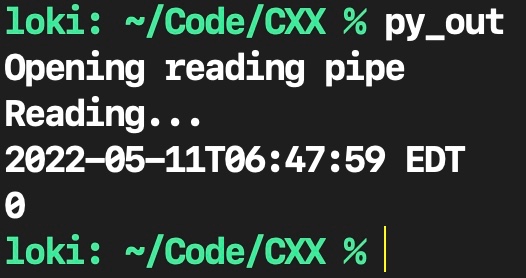